网格和布局#
Bokeh 包括用于绘图和小部件的多种布局选项。这些选项使您可以排列多个组件以创建交互式仪表板和数据应用程序。
布局函数使您可以构建绘图和小部件的网格。您可以在一个布局中拥有任意多行、列或绘图网格。Bokeh 布局还允许使用多种尺寸调整选项或模式。这些模式允许绘图和小部件调整大小以适应浏览器窗口。
内置布局#
列布局#
要垂直显示绘图或小部件,请使用 column()
函数。
from bokeh.layouts import column
from bokeh.plotting import figure, show
x = list(range(11))
y0 = x
y1 = [10 - i for i in x]
y2 = [abs(i - 5) for i in x]
# create three plots
s1 = figure(width=250, height=250, background_fill_color="#fafafa")
s1.scatter(x, y0, size=12, color="#53777a", alpha=0.8)
s2 = figure(width=250, height=250, background_fill_color="#fafafa")
s2.scatter(x, y1, size=12, marker="triangle", color="#c02942", alpha=0.8)
s3 = figure(width=250, height=250, background_fill_color="#fafafa")
s3.scatter(x, y2, size=12, marker="square", color="#d95b43", alpha=0.8)
# put the results in a column and show
show(column(s1, s2, s3))
行布局#
要水平显示绘图或小部件,请使用 row()
函数。
from bokeh.layouts import row
from bokeh.plotting import figure, show
x = list(range(11))
y0 = x
y1 = [10 - i for i in x]
y2 = [abs(i - 5) for i in x]
# create three plots
s1 = figure(width=250, height=250, background_fill_color="#fafafa")
s1.scatter(x, y0, size=12, color="#53777a", alpha=0.8)
s2 = figure(width=250, height=250, background_fill_color="#fafafa")
s2.scatter(x, y1, size=12, marker="triangle", color="#c02942", alpha=0.8)
s3 = figure(width=250, height=250, background_fill_color="#fafafa")
s3.scatter(x, y2, size=12, marker="square", color="#d95b43", alpha=0.8)
# put the results in a row and show
show(row(s1, s2, s3))
绘图的网格布局#
使用 gridplot()
函数在网格中排列 Bokeh 绘图。此函数还会将所有绘图工具合并到单个工具栏中。然后,网格中的每个绘图都具有相同的活动工具。
您可以通过传递 None
而不是绘图对象来使网格单元格留空。
from bokeh.layouts import gridplot
from bokeh.plotting import figure, show
x = list(range(11))
y0 = x
y1 = [10 - i for i in x]
y2 = [abs(i - 5) for i in x]
# create three plots
s1 = figure(background_fill_color="#fafafa")
s1.scatter(x, y0, size=12, alpha=0.8, color="#53777a")
s2 = figure(background_fill_color="#fafafa")
s2.scatter(x, y1, size=12, marker="triangle", alpha=0.8, color="#c02942")
s3 = figure(background_fill_color="#fafafa")
s3.scatter(x, y2, size=12, marker="square", alpha=0.8, color="#d95b43")
# make a grid
grid = gridplot([[s1, s2], [None, s3]], width=250, height=250)
show(grid)
为方便起见,您也可以只传递绘图列表并指定网格中所需的列数。例如
gridplot([s1, s2, s3], ncols=2)
您还可以传入 width
和 height
参数。这些尺寸将应用于您的所有绘图。
默认情况下,gridplot()
会将所有子绘图工具合并到单个父网格工具栏中。要禁用此行为,请将 merge_tools
设置为 False
。
from bokeh.layouts import gridplot
from bokeh.plotting import figure, show
x = list(range(11))
y0 = x
y1 = [10 - i for i in x]
y2 = [abs(i - 5) for i in x]
# create three plots
s1 = figure(background_fill_color="#fafafa")
s1.scatter(x, y0, size=12, alpha=0.8, color="#53777a")
s2 = figure(background_fill_color="#fafafa")
s2.scatter(x, y1, size=12, marker="triangle", alpha=0.8, color="#c02942")
s3 = figure(background_fill_color="#fafafa")
s3.scatter(x, y2, size=12, marker="square", alpha=0.8, color="#d95b43")
# make a grid
grid = gridplot([s1, s2, s3], ncols=2, width=250, height=250)
show(grid)
通用网格布局#
您可以使用 layout()
函数将绘图和小部件排列到网格中。这会自动生成适当的 row()
和 column()
布局,从而节省您的时间。尝试以下代码以查看此函数的工作方式。
sliders = column(amp, freq, phase, offset)
layout([
[bollinger],
[sliders, plot],
[p1, p2, p3],
])
这将产生以下布局
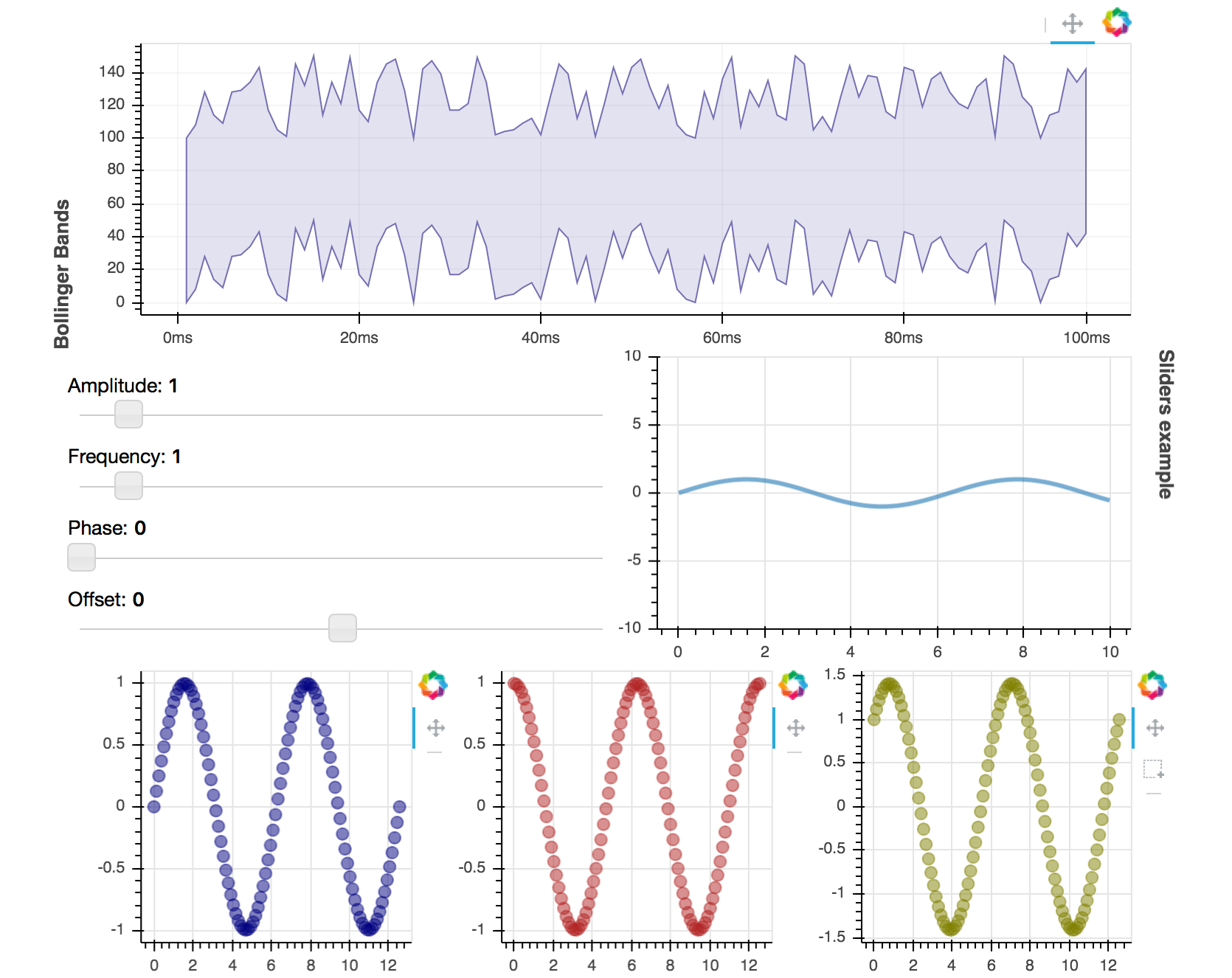
有关完整代码,请参见 examples/basic/layouts/dashboard.py。
尺寸调整模式#
模式#
使用以下尺寸调整模式来配置 Bokeh 对象在布局中的行为
"fixed"
组件保持其宽度和高度,而与浏览器窗口大小无关。
"stretch_width"
组件调整大小以填充可用宽度,但不保持任何纵横比。高度取决于组件类型,并且可以适合其内容或固定。
"stretch_height"
组件调整大小以填充可用高度,但不保持任何纵横比。宽度取决于组件类型,并且可以适合其内容或固定。
"stretch_both"
组件调整大小以填充可用宽度和高度,但不保持任何纵横比。
"scale_width"
组件调整大小以填充可用宽度,并保持原始或指定的纵横比。
"scale_height"
组件调整大小以填充可用高度,并保持原始或指定的纵横比。
"scale_both"
组件调整大小以填充可用宽度和高度,并保持原始或指定的纵横比。
根据模式,您可能还需要指定 width
和/或 height
。例如,使用 stretch_width
模式时,您必须指定固定高度。
单个对象#
下面的示例允许您从下拉列表中选择尺寸调整模式,并查看单个绘图如何响应不同的模式。
注意
如果封闭的 DOM 元素未定义任何要填充的特定高度,则缩放或拉伸到高度的尺寸调整模式可能会将您的绘图缩小到最小尺寸。
多个对象#
下面是一个更复杂但相当典型的嵌套布局示例。
在此布局中,子组件包括具有不同尺寸调整模式,如下所示
# plot scales to original aspect ratio based on available width
plot = figure(..., sizing_mode="scale_width")
# slider fills all space available to it
amp = Slider(..., sizing_mode="stretch_both")
# fixed sized for the entire column
widgets = column(..., sizing_mode="fixed", height=250, width=150)
# heading fills available width
heading = Div(..., height=80, sizing_mode="stretch_width")
# entire layout fills all space available to it
layout = column(heading, row(widgets, plot), sizing_mode="stretch_both")
局限性#
Bokeh 布局系统不是通用的布局引擎。它有意牺牲了一些功能,以使常见的用例和场景易于表达。具有许多不同尺寸调整模式的复杂布局可能会产生不良结果,无论是在性能还是视觉外观方面。对于更复杂的设计,请结合您自己的自定义 HTML 模板使用 网页 中提供的方法。这将使您可以利用更复杂的 CSS 布局可能性。